Quick Search
FlexAdmin allows you to search across all your resources using the Quick search input located within the left navigation bar of the FlexAdmin administration panel:
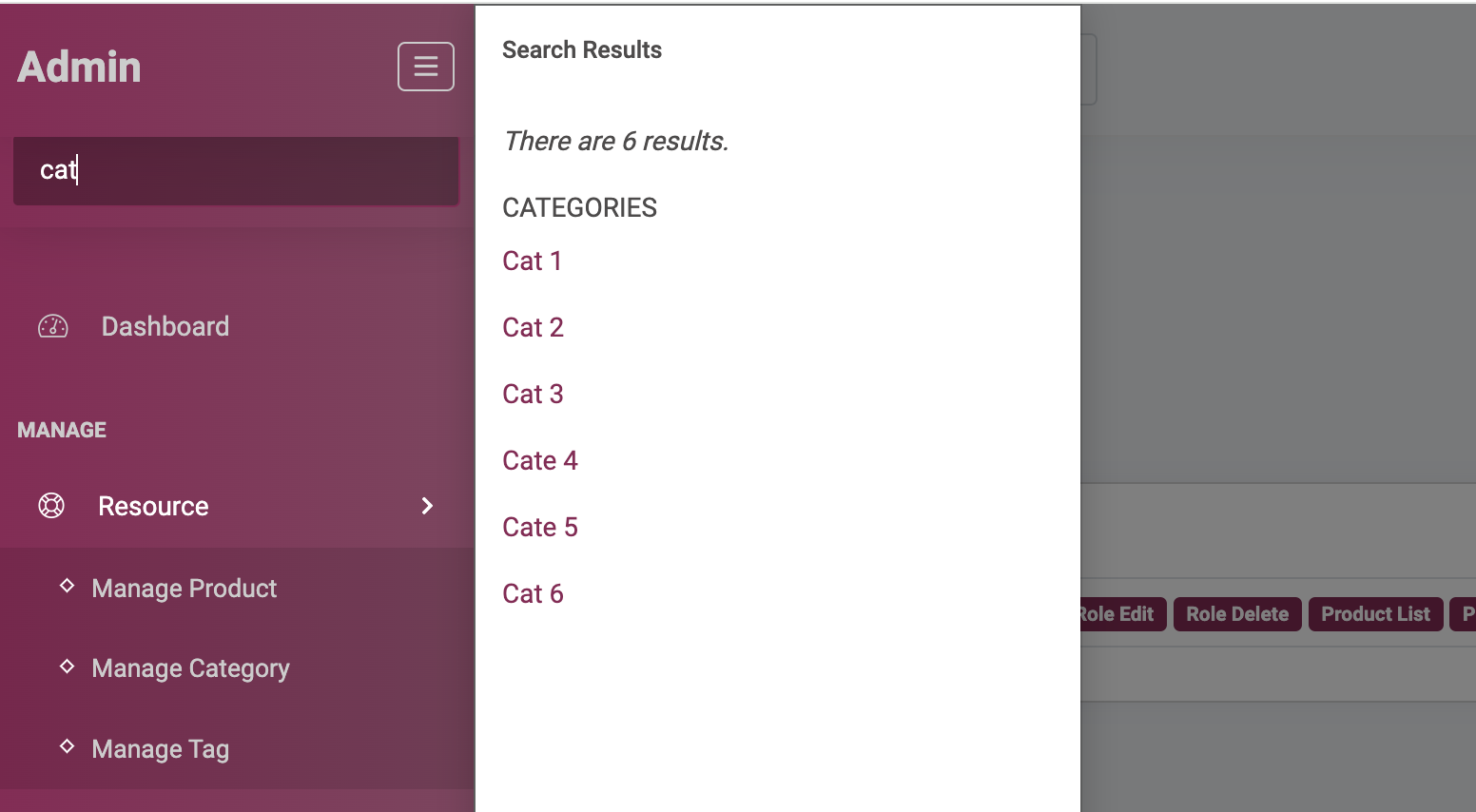
Customization
Preparing your models
To search through models you’ll have to let them implement the Searchable
interface.
namespace Spatie\Searchable;
interface Searchable
{
public function getSearchResult(): SearchResult;
}
You’ll only need to add a getSearchResult
method to each searchable model that must return an instance of SearchResult
. Here’s what it could look like for a blog post model.
use Spatie\Searchable\Searchable;
use Spatie\Searchable\SearchResult;
class BlogPost extends Model implements Searchable
{
public function getSearchResult(): SearchResult
{
// $url = route('blogPost.show', $this->slug);
$url = route('blogPost.show', $this->id);
return new \Spatie\Searchable\SearchResult(
$this,
$this->title,
$url
);
}
}
Register the new SearchModel
Go to app/Http/Controllers/Admin/QuickSearchController.php
file
Add new line:
->registerModel(BlogPost::class, 'name')
public function search(Request $request)
{
$searchResults = (new Search())
->registerModel(Category::class, 'name')
->registerModel(BlogPost::class, 'name') // Register your Model here
->search($request->input('query'));
return view('admin.quick-search.search-result', compact('searchResults'));
}
Limiting aspect results
Go to app/Http/Controllers/Admin/QuickSearchController.php
file
Add the limitAspectResults number
public function search(Request $request)
{
$searchResults = (new Search())
->registerModel(Category::class, 'name')
->registerModel(BlogPost::class, 'name')
->limitAspectResults(5) // Add the limit aspect results here
->search($request->input('query'));
return view('admin.quick-search.search-result', compact('searchResults'));
}
Javacsript file:
resources/js/admin/quick_search.js